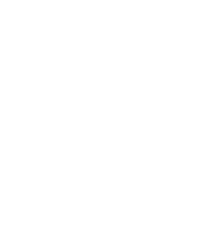
CompSci 201: Data Structures & Algorithms
Recitation
Fall 2012
Recitation 4:Hashing and CompareTo
September 21, 2012
In preparation for Friday's recitation, I'd like you to create a class that has a compareTo method like we used in SimpleSort.java. compareTo is also used by a lot of internal Java functions, such as Collections.sort.
Snarf the recitation 4 code. In it you'll see a ThreeInts class. This class has a constructor:
public ThreeInts(int one, int two, int three)
And, a main method that will run the code:
public static void main(String[] args)
.
However, the code won't compile because it needs a compareTo method. Your task is to add a new compareTo method:
public int compareTo(ThreeInts other) {
//your code goes here
}
The way this compareTo method works is that it compares one ThreeInts instance to another ThreeInts instance (called other). compareTo returns either a number greater than 0, less than 0, or 0...depending on which object is larger. That is, you would use compareTo like this:
ThreeInts a = new ThreeInts(1,2,3);
ThreeInts b = new ThreeInts(7,0,0);
int result = a.compareTo(b);
if(result < 0)
System.out.println("a is less than b");
if(result == 0)
System.out.println("a is equal to b");
if(result > 0)
System.out.println("a is greater than b");
For recitation, add the compareTo methods for the TheeInts class. Your compareTo should work for ThreeInts objects as follows:
If the sum of the three ints in one ThreeInts object are greater than the sum in another ThreeInts object, the first is greater than the second. For example, in the code above,
a < b
because 1 + 2 + 3 < 7 + 0 + 0
.
If you implement compareTo correctly, the sort function in the main should work and your code should print out:
[<85,-89,2>, <0,0,0>, <-5,0,7>, <0,0,100>]
Submit your code before class.